698. 划分为k个相等的子集
https://leetcode.cn/problems/partition-to-k-equal-sum-subsets/ 给定一个整数数组 nums 和一个正整数 k,找出是否有可能把这个数组分成 k 个非空子集,其总和都相等。 示例 1:
输入: nums = [4, 3, 2, 3, 5, 2, 1], k = 4
输出: True
说明: 有可能将其分成 4 个子集(5),(1,4),(2,3),(2,3)等于总和。示例 2:
输入: nums = [1,2,3,4], k = 3
输出: false提示:
- 1 <= k <= len(nums) <= 16
- 0 < nums[i] < 10000
- 每个元素的频率在 [1,4] 范围内
# 题解
class Solution { |
复杂度分析:
- 时间复杂度 \(O (n*2^n)\)
- 空间复杂度 \(O (2^n)\)
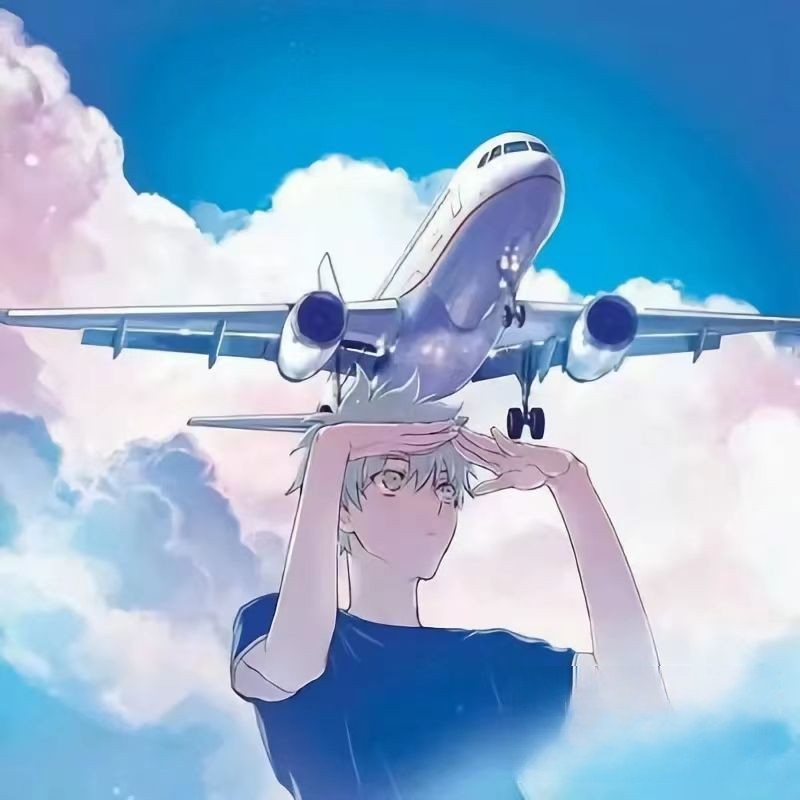
Invitation
x-17
202111170521
created:2021/11/17
Welcome to X
月缺不改光,剑折不改钢
共矜然诺心,各负纵横志
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 潇十七!
评论