854. 相似度为 K 的字符串
https://leetcode.cn/problems/k-similar-strings/ 对于某些非负整数 k ,如果交换 s1 中两个字母的位置恰好 k 次,能够使结果字符串等于 s2 ,则认为字符串 s1 和 s2 的 相似度为 k 。 给你两个字母异位词 s1 和 s2 ,返回 s1 和 s2 的相似度 k 的最小值。 示例 1:
输入:s1 = "ab", s2 = "ba"
输出:1示例 2:
输入:s1 = "abc", s2 = "bca"
输出:2提示:
- 1 <= s1.length <= 20
- s2.length == s1.length
- s1 和 s2 只包含集合 {‘a’, ‘b’, ‘c’, ‘d’, ‘e’, ‘f’} 中的小写字母
- s2 是 s1 的一个字母异位词
# 题解
class Solution { |
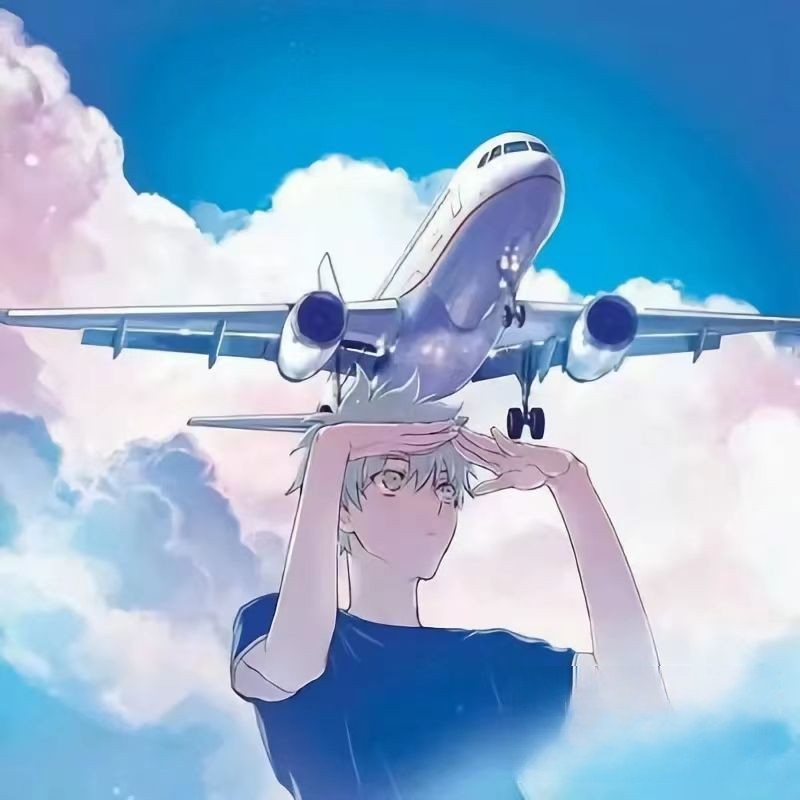
Invitation
x-17
202111170521
created:2021/11/17
Welcome to X
月缺不改光,剑折不改钢
共矜然诺心,各负纵横志
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 潇十七!
评论